Obsolescence Guaranteed
Recommended: Gigatron
a computer w/o a microprocessor
KIM Uno: a DIY clone of the KIM-1
Calculator Introduction
This page describes the calculator functions of the KIM Uno. Software archaeology is nice; 6502 programming is fun and interesting. But you have got to wonder what you are actually going to do, regularly, with something as obsolete as a KIM-1 in the 21st century...
The one useful role such technology still can perform well is to be a programmable calculator.
So the idea is to provide a 6502 programmable calculator, using the KIM-1 environment with minimal extensions. Basically, a feature to show floating point numbers with 7 digits and decimal point.
There's also normal non-programmable calculator mode (press SST for >1 second to toggle in and out of that mode). It simply changes the assignment of the keys on the KIM Uno keypad so you can enter and view floating point numbers interactively, and you should see this mode as much as a debugger for when you write calculator programs as it is a normal non-programmable calculator mode on its own.
if you think of early microcomputers as glorified calculators, it's surprising to find out just how hard it is to do simple floating point math on a CPU. Some of the very earliest programming on microprocessors - before operating systems even arrived - was thus to produce math routines. In 1976, MOS Technology published KIMATH, and Roy Rankin and Steve Wozniak published a competing math library in Dr. Dobb's Journal. In recent years, Charles R Bond has created a new 6502 math library, fltpt65, which is probably the last word in 6502 math programming. This is the one used in the KIM Uno. It's placed in a ROM starting at $5000.
​
Quick Start: The KIM as 6502 Programmable Calculator
​
There is a built-in demo program at $0210 that you can try. It asks you to enter two floating point numbers, and it then shows you the product of both. Next, it asks you for an integer number, used as a yes/no question. If you enter 00, the program ends by showing the last result again. If you enter 01, it shows the square root of the previous answer.
Enter 0210 [GO]
Enter 4 [GO]
Enter 5 [GO]
View number, then press [GO] (4*5=20.000)
Enter 01 [GO] (01 = yes, take square root)
View number, then press [GO] (sqrt(20) = 4.472135)
​
You can read the program either as hex code on the display, or use the disassembler or mini-assembler to list it on the serial port. Basically, simple calculator programs consist of
-
JSR 70Ax calls to ask for floating point numbers;
-
JSR 70xy calls to copy/swap floating point numbers between the 'registers' that store them for you;
-
LDA XX statements to choose the math operation (00=add, 02=multiply, etc, see below);
-
JSR 00 50 calls to execute calculations;
-
JSR 70Dx calls to display floating point numbers;
-
BEQ statements to do if-then statements.
​
You're pretty much set if you know just the hex codes for three 6502 instructions used above, plus the addresses of the various JSR 'Ask/Display' calls. See further below for details.
Technical interlude: fltpt65
Fltpt65 requires you to:
-
enter the two numbers you want to work with in its W1 and W2 registers,
-
enter the operation you want it to perform in the 6502's A register,
(using the KIM Monitor environment, you can put the value in $00F3, where the monitor will pick it up and store it in A for you just before starting program execution).
​
Then, you call the program at $5000 (so 5000 [GO] on the KIM), and fltpt65 will provide the result in its W3 register. There also is a W4, which you can use as a calculator memory. The four registers are 8-byte locations in memory, starting at $0360.
​
The above is simple enough. But W1 and W2 are encoded floating point numbers, not just single-byte integers, so they are hard to handle. Each W register is an array of 8 bytes that (ignore the following unless you care) represents a floating point number, BCD encoded, with 12 mantissa digits and 3 exponent digits supporting a maximum value of +9.999999999 E+999.
The picture below shows the format of fltpt65 numbers. But the point is, this format is hard to use for humans. The KIM Uno has to provide some user interface to read and write W registers.
Fltpt65 floating point format. Source: CRBond.com
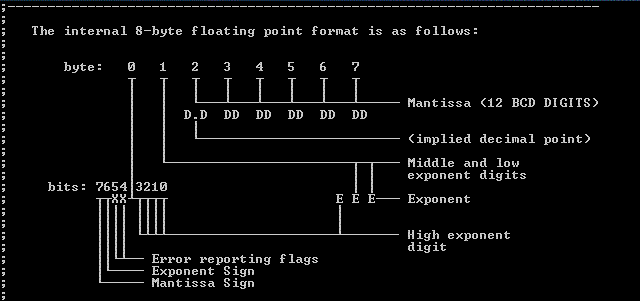
6502 Programmable Calculator:
use fltpt65 in mini KIM-1 programs
You can call the fltpt65 subroutines from a small program like the one below. Load the floating point numbers you want to work with in W1 and W2 (top two lines). Load the A register with the desired operation, and call $5000 from within your program. Then display the result of the calculation in W3.
​
This makes the KIM Uno a pretty decent programmable calculator, which you code in 6502 instructions. The code can be very simple, see the example below. Remember that you can store your code in the Eeprom (1K of extra memory starting from $0400) so nothing gets lost if you power off.
​
Code of the example program stored at $0210:
​
JSR $70A1 ; ask user for W1
JSR $70A2 ; ask user for W2
LDA #$02 ; want to multiply W1 * W2
JSR $5000 ; execute calculation
JSR $70D3 ; display W3
JSR $70AA ; ask user for integer value in A register
BEQ SKIP ; skip sqrt calculation if user entered 0
JSR $7031 ; copy result of multiplication in W3 to W1
LDA #$04 ; take square root of W1
JSR $5000 ; execute calculation
SKIP: JSR $70D3 ; display W3
BRK ; end
​
​
$7XXX Function calls for 6502 Programs:
-$70Ax: Ask user for input-------------------------
$70A1/2/3/4: Ask user to enter number in W1/2/3/4
$70AA: Ask user to enter integer into A register
-$70Dx: Display number-----------------------------
$70D1/2/3/4: Display number in W1/2/3/4
-$70xy: Copy number between registers--------------
$70xy: Copy Wx to Wy
-$71xy: Swap numbers in registers------------------
$71xy: Swap values in Wx and Wy
---------------------------------------------------
​
The function call addresses are kind of logical if you think of it this way:
-
They are collectively called the 7000 calls;
-
The (A)sk a number calls are 7-0-A-Register number
-
The (D)isplay a number calls are 7-0-D-Register number
-
The copy calls are the 7-0 series (7-0-X-to-Y)
-
The swap calls are the 7-1 series (7-1-X-with-Y)
​
Operations: load value in A before calling $5000:
---------------------------------------------
add: 00 | sin: 10 | asec: 20 | asinh: 30
sub: 01 | cos: 11 | acot: 21 | acosh: 31
mul: 02 | tan: 12 | loge: 22 | atanh: 32
div: 03 | csc: 13 | exp: 23 | acsch: 33
sqrt: 04 | sec: 14 | sinh: 24 | asech: 34
inv: 05 | cot: 15 | cosh: 25 | acoth: 35
int: 06 | asin: 16 | tanh: 26 | log2: 36
frac: 07 | acos: 17 | csch: 27 | log10: 37
abs: 08 | atan: 18 | sech: 28 | pow: 38
chs: 09 | acsc: 19 | coth: 29 |
​
When programming, don't forget that you can use disasm to view assembly code on the serial port. At least until you know the opcodes for LDA, JSR and BEQ and maybe a few more by heart... The Mass:werk online assembler is a comfortable assembler if you need one. Lastly, you may want to try the branch calculator at $01A5 if you use the BEQ opcode and want to calculate relative offsets.
​
Closing note: Fltpt65 uses page 3 ($300-3AF) and Page 1 ($100-1A0) of the KIM-1 RAM as its work registers. Using fltpt65 means any data sitting there will be destroyed. You still have the 256 bytes of page 2 ($200-2FF) available to you, plus the 2 * 64 bytes stashed in the RIOTs. That amount of memory was enough to put men on the moon... You also have the 1K eeprom from $400-$800. That'll bring you to Mars. Easily.
Quick Start: Simple Calculator Mode
You can also used the KIM Uno as a regular calculator - to do 1+2=3. But it takes a bit of time to get used to the way it works. Here is a test run of the simple Calculator Mode - let's add 123 + 456:
​
Press SST for 1 second to enter Calculator Mode
Press C, 123, D, 456, F, 00, GO, PC --> Your answer is 579
​
More precisely, to use the KIM Uno as just a simple calculator:
-
Press SST for a second. The display flashes & keyboard definitions are changed to Calculator Mode.
-
Press C to enter your first number (stored in W1). Because the KIM's keyboard is not exactly a standard calculator keyboard, the following keys are redefined:
-
A--> decimal point
-
B --> use scientific notation: adds 'E' as in Exponent. So 1A25B3 is 1.25e3 is 125.
-
+ --> toggle to minus. Yes, the plus key means minus. I found that funny but intuitive, KIM style.
-
GO --> Enter the number; ST --> Cancel. Both return you to the KIM display. Or, alternatively:...
-
-
Press D to enter your second number, stored in W2.
-
Press F to enter the operation number (calculation) you want to do. 00 is add, 01 subtract, 02 multiply.
-
See the table further down for all 38 operations you can do.
-
-
Press GO straight after and the fltpt65 library will be run. Press PC to view the result in W3.
Simple access to the W registers
If you have made any sense of the above example, you see that the KIM Uno provides keystrokes (C and D) that allow you to simply enter and read numbers in the W registers, forgetting about their underlying complexity. That is - basically - what is called the Calculator mode: you briefly step out of the emulated KIM-1 to enter or display, one of the W registers. Once you've entered the number, or you've seen it, you're dropped back into the KIM-1 so fltpt65 can do its calculations.
​
It's a weird way to operate a calculator, but it can be useful in debugging programs for the programmable calculator too: you can edit and inspect the W registers at any time by switching into the Calculator mode by depressing SST for 1 second.
​
Whilst you're looking at W registers, you'll notice that you have 7 LED digits and, of course, decimal points between them. The moment you drop back into the KIM, you revert to the original 6-digit KIM-1 display.
Calculator keystrokes: Using Simple Calculator Mode
Enter Calculator Mode by pressing the SST key for >1 second. The keyboard now switches into Calculator Mode, meaning the keyboard gets redefined as per the tables below. To exit Calculator Mode, press SST again for >1 second.
Entering & Viewing numbers:
---------------------------------------------------
C - Enter value of W1 [AD] - View value of W1
D - Enter value of W2 [DA] - View value of W2
[PC] - View value of W3
F - Enter desired operation
---------------------------------------------------
When you are entering a number by pressing C or D:
---------------------------------------------------
A - Decimal point
B - Add exponent (for scientific notation)
+ - Toggle sign. Yes, plus means minus!
---------------------------------------------------
GO- Store number and return to KIM
ST- Cancel and return to KIM
D - Store number, continue with entering second nr
F - Store number, continue with entering operation
---------------------------------------------------
​
Operations that can be entered after pressing F:
---------------------------------------------------
add: 00 | sin: 10 | asec: 20 | asinh: 30
sub: 01 | cos: 11 | acot: 21 | acosh: 31
mul: 02 | tan: 12 | loge: 22 | atanh: 32
div: 03 | csc: 13 | exp: 23 | acsch: 33
sqrt: 04 | sec: 14 | sinh: 24 | asech: 34
inv: 05 | cot: 15 | cosh: 25 | acoth: 35
int: 06 | asin: 16 | tanh: 26 | log2: 36
frac: 07 | acos: 17 | csch: 27 | log10: 37
abs: 08 | atan: 18 | sech: 28 | pow: 38
chs: 09 | acsc: 19 | coth: 29 |
---------------------------------------------
After entering the two-digit number,
the KIM's PC is set to 5000 for you, so
just press [GO] to run the calculation and
then press [PC] to view result in W3
---------------------------------------------
​
That's basically it for the non-programmable calculator mode. Keep in mind: it acts just as much as an interactive debugger/number viewer/number editor when writing 'programmable calculator' programs.